AI Movie Generator
AI Movie Generator was my senior capstone project that was completed from September - December 2022. I developed and designed a website that generates hypothetical movie details through use of Artificial Intelligence. I utilized OpenAI’s API to access GPT-3 and DALL-E.
Context
This website was designed with human and AI co-creativity in mind, allowing users to input the actors, director, genre, setting, and a random object that will affect the results. With this information, the website is able to generate a movie page with all the necessary information as well as images from the three acts of the hypothetical film. By providing users with the tools to quickly create entertaining and humorous movie pages, I hope to provide an exciting and engaging new way for movie fans to experience the creative process behind films.
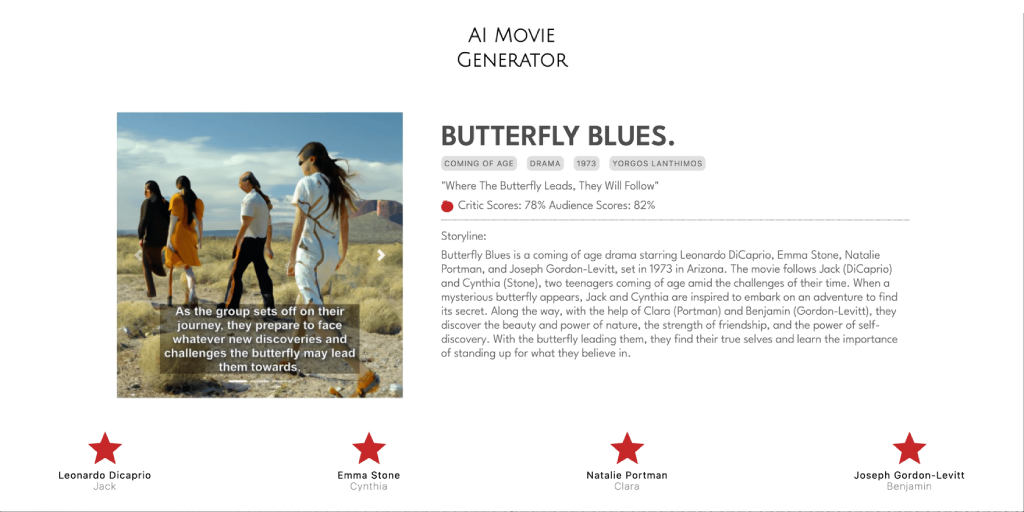
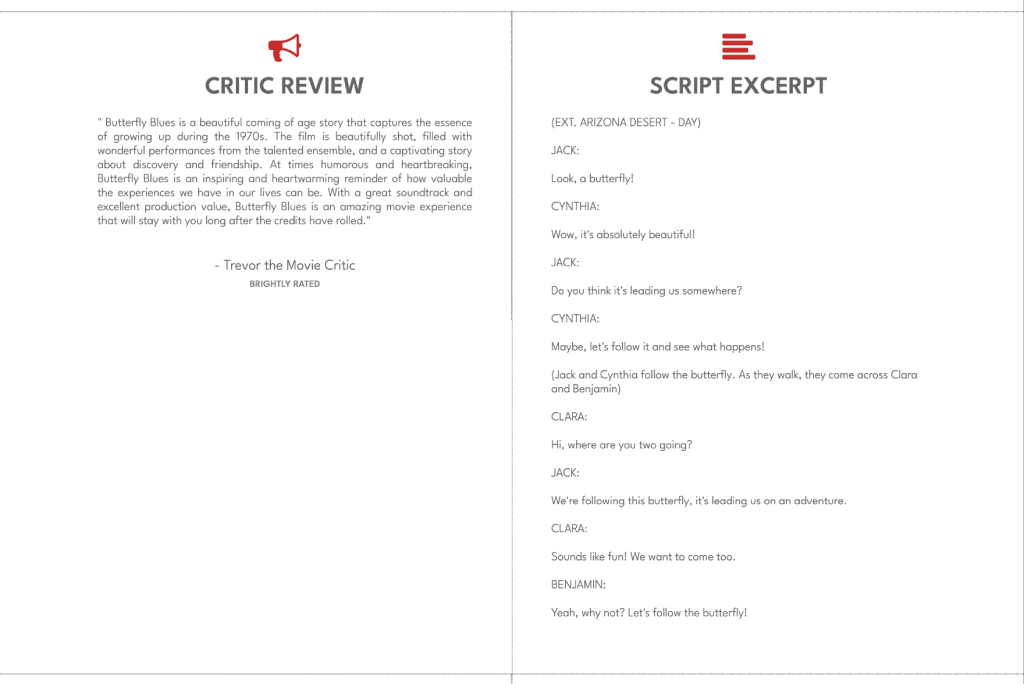

Documentation Video
The process
Initial Open AI & API Use
I began this project by testing OpenAI’s API within my computer’s terminal using python. I initially input two actors names and received a movie title, tagline, and plot summary as outputs.
Below is an example code snippet of a request to get a movie title after receiving two inputs (actor1 and actor2).
response = openai.Completion.create(
model="text-davinci-003",
prompt="Write a movie title for a coming of age romantic comedy starring "+ actor1 +" and "+ actor2 +" directed by Judd Apatow",
temperature=0.7,
max_tokens=256,
top_p=1,
frequency_penalty=0,
presence_penalty=0
)
Here is the response from the AI model given that actor1 = Mark Ruffalo and actor2 = Jennifer Garner:
Movie Title:
The Life and Times of Mark Ruffalo and Jennifer Garner
Flask App
Now that I got the API to work with inputs from the user, I got to work on creating a Flask app.
Flask is a web application framework written in Python. Flask is based on the Werkzeg WSGI toolkit and the Jinja2 template engine. (pythonbasics.org)
Jinja2 allowed me to easily pass in Python variables to my HTML file.
I am able to define a variable for the movie title, called “this_title”, and pass that into the HTML webpage as shown below
<h1>{{this_title}</h1>
↗ This video was helpful for getting started with the Flask app
Beginnings of Bootstrap Styling
As I began to consider styling for both the entry and main pages of the website, I came across Bootstrap as a resource. Bootstrap is a popular CSS framework for developing responsive sites. Bootstrap was a helpful tool to work with as I got more comfortable with front-end web development.
Basic Bootstrap styling for the input page
Basic Bootstrap styling for movie information page
Adjusting Code
You’ll notice that at this point I am not getting the best outputs and in some cases (like the movie review in the above case), my response is not generating. This was due to a couple reasons:
I was not following the best practices for prompt writing by not providing examples to the AI of the output that I wanted and
I was using the Davinci 2 model.
A few weeks into this project OpenAI upgraded their Davinci 2 model to Davinci 3 which improved the quality of writing and can handle more complex instructions.
This is what my prompt for title generation looked like before providing examples:
response_title = openai.Completion.create(
model="text-davinci-002",
prompt="write a movie title for a "+ response_subgenre.choices[0].text +" " +genre+" movie starring "+actor1+" and "+actor2+" directed by "+director+" set in "+date+" in "+place +" featuring a "+ robj,
temperature=0.9,
max_tokens=256,
top_p=1,
frequency_penalty=0,
presence_penalty=0
)
And this is what my prompt for title generation looks like after providing examples:
response_title = openai.Completion.create(
model="text-davinci-003",
prompt="Create a movie title for a slasher horror movie starring gemma chan and george clooney directed by Rian Johnson set in the civil war in connecticut featuring a breath mint: Mint Julep Murder\n\nCreate a movie title for a spaghetti western movie starring ralph fiennes and tommy wiseau directed by Martin Scorsese set in 2003 in Chicago featuring a elevator: The Good, the Bad, and the Vertical\n\nCreate a movie title for a slasher horror movie starring zach braff and jennifer lawrence directed by Christopher Nolan set in 1920 in New Orleans featuring a cupcake: The Sweetest Revenge \nCreate a movie title for a "+ response_subgenre.choices[0].text +" " +genre+" movie directed by "+director+" starring "+actor1+" as "+a1char+" and "+actor2+" as "+a2char+" with supporting actors "+supportingactor1+" as "+sa1char+" and "+supportingactor2+" as "+sa2char+". The movie is set in "+date+" in "+place +" featuring a "+ robj +":",
temperature=0.9,
max_tokens=256,
top_p=1,
frequency_penalty=0,
presence_penalty=0
)
Further Development
I continued to work on the visual feel of the website and the development of more prompts in tandem. I added more responses like supporting actors, on-set conflicts, movie theme, and so on. It was also at this point that I added buttons to generate a new response or to begin the process over again with new inputs.
Final Website
Acknowledgments
Thanks to Caleb Kicklighter for his guidance and feedback throughout the duration of this project.